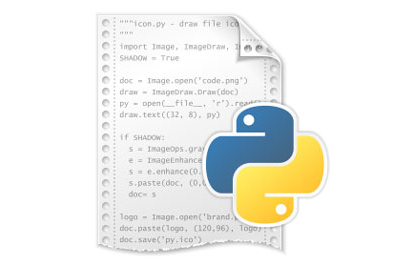
Writing scripts in python
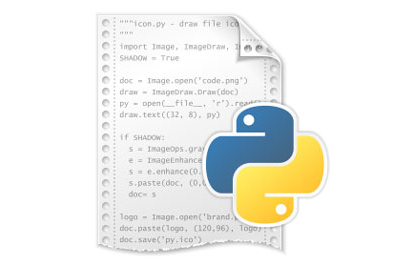
Information
The estimated time to complete this training module is 2h.
The prerequisites to take this module are:
- the installation module.
Contact Hao-Ting Wang if you have questions on this module, or if you want to check that you completed successfully all the exercises.
Resources
This module was presented by Greg Kiar during the QLSC 612 course in 2020, with slides from Joel Grus’ talk at JupyterCon 2018.
The video is available below:
Exercise
In this exercise we will program a key-based encryption and decryption system. We will implement a version of the Vigenere cipher, but instead of just using the 26 letters of the alphabet, we will use all the unicode characters.
The Vigenere cipher consists in shifting the letters of the message to encrypt by the index of the corresponding letter in the key. For example the encryption of the letter B with the key D will result in the letter of new_index = index(B) + index(D) = 2 + 4 = 6, so it will be the 6th letter which is F.
⚠ Note that here by index I mean the index of the letter in the alphabet and not the index of the letter in the string.
You pair up the letters of the message with the ones of the key one by one, and repeating the key if it is shorter than the message. For example if the message is message
and the key is key
, the pairs will be :
(m,k),
(e,e),
(s,y),
(s,k),
(a,e),
(g,y),
(e,k)
For the indices of the letter, we will not use the the number of the letter in the alphabet, but the unicode index of the letter, which is easily obtained with the native python function ord
. The reverse operation of getting a letter from its unicode index is obtained with native python function chr
. There are 1114112 unicode characters handled by python, so we'll have to make sure we have indices in the range 0 to 1114111. To ensure that, we can use values modulo 1114112, i.e. encrypted_index = (ord(message_letter) + ord(key_letter)) % 1114112
.
Step 1: Create relevant functions in useful_functions.py
You'll implement the following functions :
encrypt_letter(letter, key)
: return the encrypted letter with the key, e.g.encrypt_letter("l", "h")
should return'Ô'
.decrypt_letter(letter, key)
: return the decrypted letter with the key, e.g.decrypt_letter("Ô", "h")
should return'l'
.process_message(message, key, encrypt)
: return the encrypted message using the letters inkey
if encrypt isTrue
, and the decrypted message if encrypt isFalse
. For example :
process_message('coucou', 'clef', True)
'ÆÛÚÉÒá'
process_message('ÆÛÚÉÒá', 'clef', False)
'coucou'
After creating these function, try to call them in your python terminal or in a JupyterNotebook to try things out. Are the functions performing as you expected?
If so, let's make sure they are in a file named useful_functions.py
and conclude the first part of the exercise.
Step 2: Create a file cypher_script.py
:
- use the Argparse library introduced in the video so that a user can call the script with three arguments :
--message
,--key
and--mode
.--message
will contain the path to a text files containing the message.--key
will be a string directly containing the key.--mode
will be a string that can take the value"enc"
or"dec"
to tell the script if you want to encrypt or decrypt the message. - The script should import the functions from
useful_functions.py
and use them in its main function to encrypt or decrypt the text in the message file using the text in the key file as the key, and save the results in a file that has the same name as the message file but with a_encrypted
or_decrypted
suffix depending on the mode. So callingpython cypher_script.py --message msg_file.txt --key my_key --mode enc
should create amsg_file_encrypted.txt
file. - Don't forget to write the code under
if __name__ == "__main__"
. Even though in this example it won’t make a difference, it is never too early to get used to good practices. Read this section below to understand the usefulness ofif __name__ == "__main__"
.
On the usefulness of "if __name__ == '__main__':" (click to show ⬇)
It is not obvious why you should put the if __name__ == "__main__":
line in your script. Indeed in a lot of cases, putting it or not won't change anything to how your code runs. But in specific settings with multiple scripts importing from each pother, not putting it in can quickly lead to a nightmare.
To give you an insight of how and why it is useful, here is an example (if you don't want to read or if you want complementary explanations, here is a nice youtube video about it).
Suppose you have a script to fit a Ridge model on provided data, judiciously named fit_Ridge.py
, which looks like this :
#!/usr/bin/env python
import argparse
import pickle # pickle is a librairie to save and load python objects.
import numpy as np
from sklearn.linear_model import Ridge
def fit_Ridge_model(X, Y):
model = Ridge()
model.fit(X, Y)
return model
parser = argparse.ArgumentParser()
parser.add_argument("--X_data_path", type=str)
parser.add_argument("--Y_data_path", type=str)
parser.add_argument("--output_path", type=str)
args = parser.parse_args()
X = np.load(args.X_data_path)
Y = np.load(args.Y_data_path)
model = fit_Ridge_model(X, Y)
pickle.dump(model, open(args.output_path, 'wb'))
This script allows the user to provide the paths to two numpy files as data to fit a Ridge model, and to save the model to the provided path with a command like :
python fit_Ridge.py --X_data_path data_folder/X.npy --Y_data_path data_folder/Y.npy --output_path models/Ridge.pk
There is no if __name__ == "__main__":
to be seen but, used on its own, the script works fine.
But later, you write an other script compare_to_Lasso.py
that compare Ridge and Lasso models on the same data, so you need to fit a Ridge model again. Eager to apply the good practices of programming, you judiciously decide not to duplicate the code for fitting a ridge model, but to import the fit_Ridge_model
function from the fit_Ridge.py
. Thus your second script looks like that :
#!/usr/bin/env python
import numpy as np
import argparse
from sklearn.linear_model import Lasso
from fit_Ridge import fit_Ridge_model
parser = argparse.ArgumentParser()
parser.add_argument("--X_data_path", type=str)
parser.add_argument("--Y_data_path", type=str)
args = parser.parse_args()
X = np.load(args.X_data_path)
Y = np.load(args.Y_data_path)
ridge_model = fit_Ridge_model(X, Y)
lasso_model = Lasso()
lasso_model.fit(X, Y)
ridge_score = ridge_model.score(X, Y)
lasso_score = lasso_model.score(X, Y)
if Ridge_score > lasso_score:
print("Ridge model is better.")
else:
print("Lasso model is better.")
It seems fine but here when you try to call
python compare_to_Lasso.py --X_data_path data_folder/x.npy --Y_data_path data_folder/Y.npy
you get an error :
Traceback (most recent call last):
File "compare_lasso_ridge.py", line 5, in <module>
from fit_Ridge import fit_Ridge_model
File "/Users/francois/scratch/fit_Ridge.py", line 21, in <module>
pickle.dump(model, open(args.output_path, 'wb'))
TypeError: expected str, bytes or os.PathLike object, not NoneType
The error shows that the script tried to save a model to the path args.output_path
, which was not defined so it was set to None and raised a TypeError. But our compare_to_Lasso.py
script never tries to save a model ! Indeed looking at the other lines of the error message, we see that it comes from the import. In fact what happens is that when we try to import the fit_Ridge_model
function from the fit_Ridge.py
file, python will read the entire file and execute everything that is written in it, so it will try to fit a Ridge model and to save it. But we don't want python to execute everything, we just want it to read the definition of the fit_Ridge_model
function. That is why here we absolutely need the if __name__ == "__main__":
, so we modify the fit_Ridge.py
script like that :
#!/usr/bin/env python
import argparse
import pickle # pickle is a librairie to save and load python objects.
import numpy as np
from sklearn.linear_model import Ridge
def fit_Ridge_model(X, Y):
model = Ridge()
model.fit(X, Y)
return model
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument("--X_data_path", type=str)
parser.add_argument("--Y_data_path", type=str)
parser.add_argument("--output_path", type=str)
args = parser.parse_args()
X = np.load(args.X_data_path)
Y = np.load(args.Y_data_path)
model = fit_Ridge_model(X, Y)
pickle.dump(model, open(args.output_path, 'wb'))
Now when importing from this script, python will read the definition of the function, but after that it will not execute the rest, since during the import the variable __name__
is not set to "__main__"
but to "fit_Ridge"
.
In the end using if __name__ == "__main__":
is the only way to safely import functions from our script, and since you never know for sure that you won't have to import something from a script in the future, putting it in all of your script by default is not a bad idea.
Step 3: verify your results
Finally, decrypt the file obtained with :
wget https://raw.githubusercontent.com/BrainhackMTL/psy6983_2021/master/content/en/modules/python_scripts/message_encrypted.txt
with the following key :
my_super_secret_key
- Follow up with Hao-Ting Wang to validate you completed the exercise correctly.
- 🎉 🎉 🎉 you completed this training module! 🎉 🎉 🎉
More resources
If you are curious to learn more advanced capabilities for the Argparse library, you can check this Argparse tutorial.
To learn more about python in general, you can check the tutorials of the official python documentation and choose the topic you want to learn. I also recommend the porgramiz tutorials which have nice videos. Finally for even nicer and fancier videos there is the excellent python programming playlist from the youtube channel Socratica.